Tricks to avoid confusing environments when testing
When I started developing software for a living (around 2005), I made many mistakes, such as dropping the production database, restoring the test database to production, creating a backup, and receiving a backup from a coworker on USB, which, in turn, I also overwrote. And I did all of that within 20 minutes. We could only recover because my coworker noticed my chaotic behavior and decided to copy the database from the USB before unplugging and handing it over. 😅
Since then, I have colored my connection strings and grouped my remote desktop connections. Sometimes, I even instruct the connection string to be read-only or encrypt the file so I can't make a mistake.
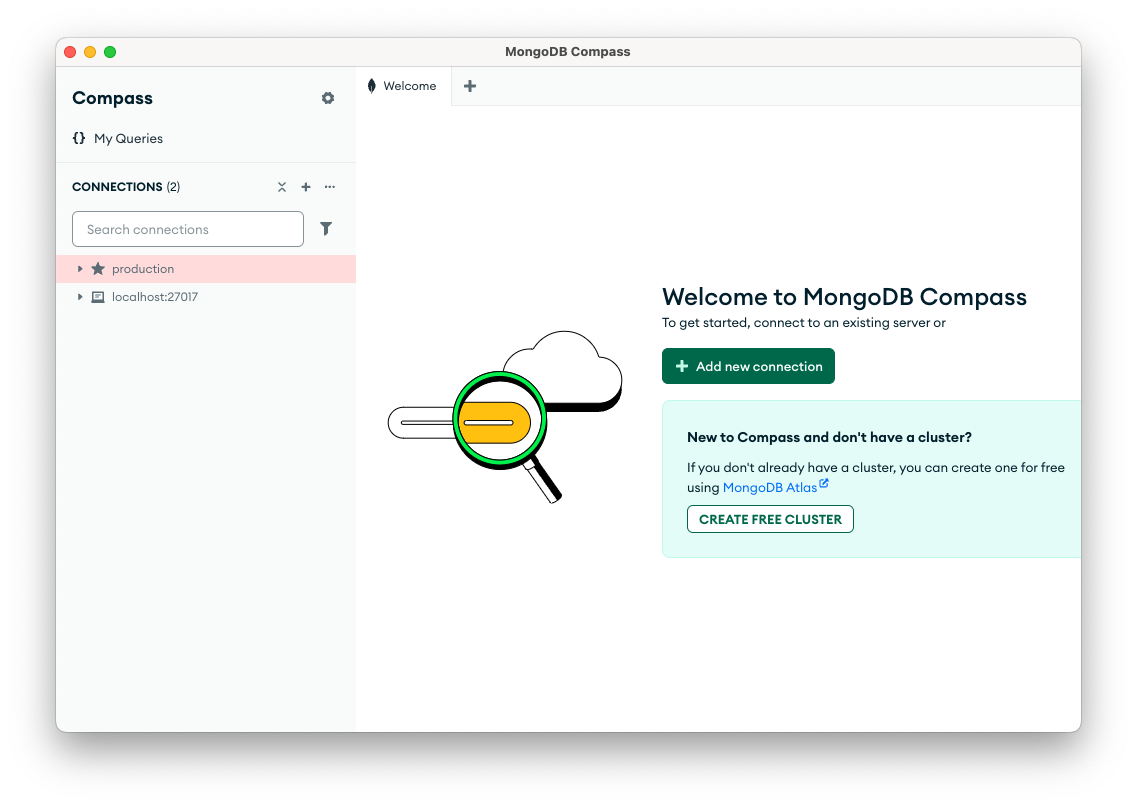
However, this doesn't work when I'm testing the product—or so you would think. One of my favorite tricks is to write a small bit of Javascript that examines the domain and introduces a variable.
// Somewhere in my application
const host = window.location.hostname;
const search = window.location.search;
var env = 'prod';
if (host == 'localhost') env = 'dev';
if (host == 'test.example.com') env = 'test';
if (search.contains('fake-prod')) env = 'prod';
if (search.contains('fake-dev')) env = 'dev';
if (search.contains('fake-test')) env = 'test';
const colorTable = {
'dev': 'red',
'test': 'blue',
'prod': undefined
};
const color = colorTable[env];
if (color) {
document.body.style
.setProperty('--background-header-color', color);
}
And in my CSS, I have the following code:
.header {
/* use the css-variable, otherwise use the next value */
background-color: var(--background-header-color, blue);
}
This way, when testing or running the app, I know precisely which environment I'm working on based on the color.
Well, except for that one time when somebody saw the different colors and preferred the color scheme of the test environment.